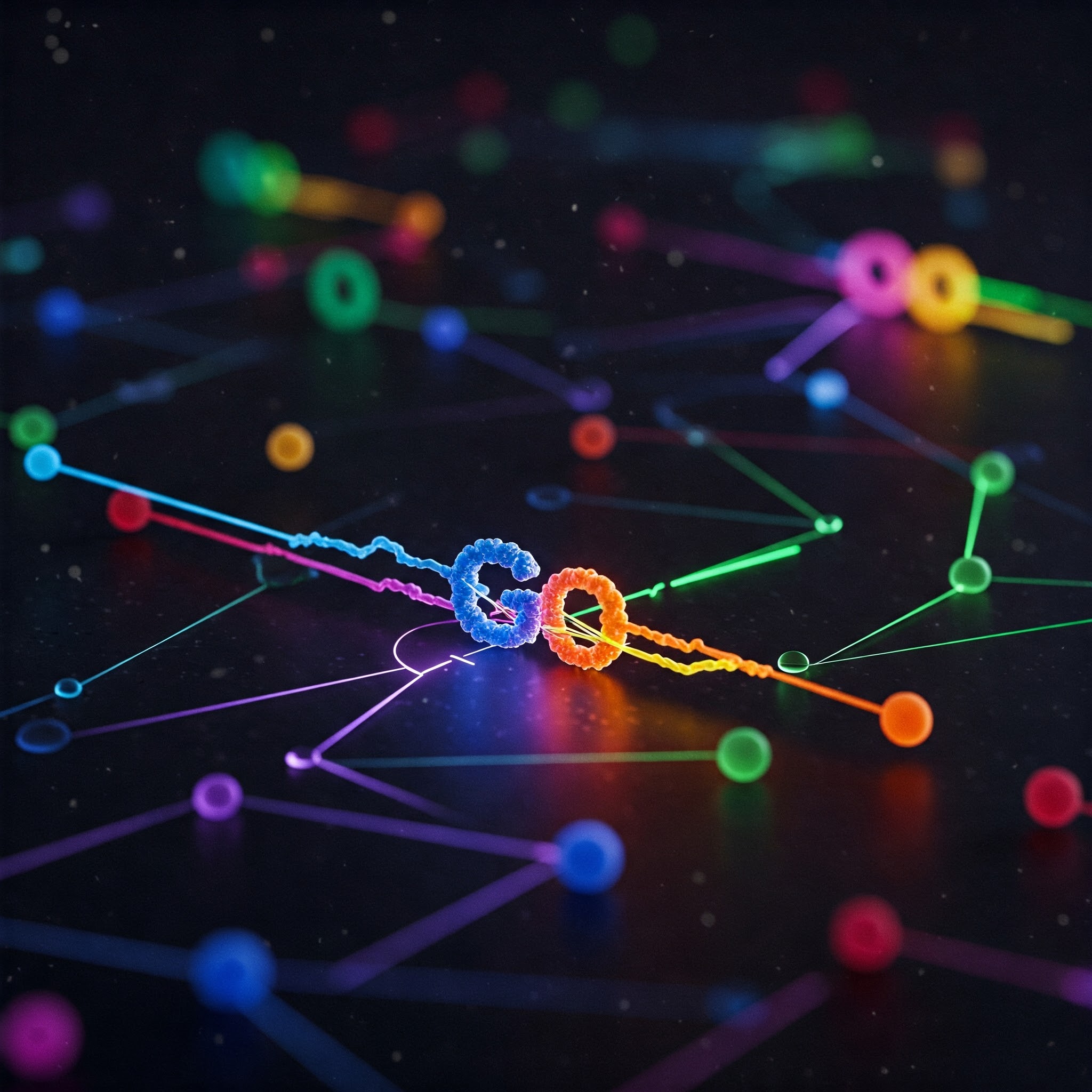
Understanding Go Channels: A Simple Guide with Kitchen Analogies
Admin • April 1, 2025
In Go (Golang), a channel is like a pipe or a queue that lets different parts of your program (called goroutines) talk to each other safely. Imagine it as a way to send and receive messages between two workers without them stepping on each other’s toes.
Here’s how it works in simple terms:
Sending and Receiving: One goroutine can "send" data into the channel, and another goroutine can "receive" that data from the channel. It’s like passing a note through a tube.
Synchronization: Channels help keep things in order. If one goroutine tries to send something but no one’s ready to receive it, the sender waits. If someone’s waiting to receive but nothing’s been sent, the receiver waits. This prevents chaos!
Example: Think of a restaurant kitchen. The chef (goroutine 1) puts a finished dish (data) into a serving window (channel). The waiter (goroutine 2) picks it up from the window to serve it. The chef doesn’t throw the dish out unless the waiter’s ready, and the waiter doesn’t grab air if there’s no dish yet.
Basic Code Example
package main
import "fmt"
func main() {
// Create a channel (like setting up the pipe)
ch := make(chan string)
// Start a goroutine to send a message
go func() {
ch <- "Hello!" // Send "Hello!" into the channel
}()
// Receive the message from the channel
msg := <-ch
fmt.Println(msg) // Prints "Hello!"
}
How It Works
- Unbuffered Channel: Like the example above, it waits for both sender and receiver to be ready (synchronous).
- Buffered Channel: You can make a channel with a size (e.g.,
make(chan string, 2)
), so it can hold some data before waiting—like a small queue. - Direction: You can specify if a channel is only for sending (
chan<-
) or receiving (<-chan
) to make your code safer.
Kitchen Analogies Code Example
Let’s implement a Go program that uses channels with your restaurant kitchen analogy—where a chef sends a dish through a serving window (channel) and a waiter receives it. We’ll make it synchronous (unbuffered channel), so the chef waits until the waiter is ready to pick up the dish, and the waiter waits until the chef has something ready.
Here’s the code:
package main
import (
"fmt"
"time"
)
func main() {
// Create an unbuffered channel (the serving window)
servingWindow := make(chan string)
// Chef goroutine: Prepares a dish and sends it to the serving window
go func() {
fmt.Println("Chef: Starting to prepare the dish...")
time.Sleep(2 * time.Second) // Simulate cooking time
dish := "Spaghetti"
fmt.Println("Chef: Dish is ready, placing", dish, "in the serving window.")
servingWindow <- dish // Send the dish to the channel (waits if no one’s ready)
fmt.Println("Chef: Dish has been picked up, back to work!")
}()
// Waiter goroutine: Waits to receive the dish from the serving window
go func() {
fmt.Println("Waiter: Waiting for a dish at the serving window...")
dish := <-servingWindow // Receive the dish from the channel (waits if nothing’s there)
fmt.Println("Waiter: Got", dish, "from the serving window, serving it now!")
}()
// Give the goroutines time to finish (in a real app, you'd use sync.WaitGroup)
time.Sleep(3 * time.Second)
fmt.Println("Shift is over!")
}
How It Works
Serving Window (Channel):
servingWindow := make(chan string)
creates an unbuffered channel. It’s like the narrow window between the kitchen and the dining area—only one dish fits at a time, and someone has to pick it up before the next one goes through.Chef (Sender): The chef goroutine prepares a "Spaghetti" dish and sends it to the
servingWindow
. If the waiter isn’t ready, the chef waits (blocked) until the waiter picks it up.Waiter (Receiver): The waiter goroutine waits at the
servingWindow
to receive a dish. If the chef hasn’t put anything there yet, the waiter waits (blocked) until a dish arrives.Synchronization: Because the channel is unbuffered, the chef and waiter sync up perfectly. The chef won’t move on until the waiter takes the dish, and the waiter won’t move on until there’s a dish to take.
Output (Example Run)
Chef: Starting to prepare the dish...
Waiter: Waiting for a dish at the serving window...
Chef: Dish is ready, placing Spaghetti in the serving window.
Waiter: Got Spaghetti from the serving window, serving it now!
Chef: Dish has been picked up, back to work!
Shift is over!
Why It’s Synchronous
- The
time.Sleep(2 * time.Second)
in the chef’s goroutine simulates cooking time. The waiter might get to the window first and wait. - When the chef sends
Spaghetti
withservingWindow <- dish
, it blocks until the waiter does<-servingWindow
. - Once the waiter picks up the dish, both goroutines can continue.
This matches the analogy: the chef doesn’t toss out the dish unless the waiter’s there, and the waiter doesn’t grab nothing—they sync up through the serving window (channel)!
Key Points
In short, channels are Go’s way of letting goroutines share data and coordinate without messing up. It’s simple but powerful!